Laravel Migrations are essential for managing database changes within Laravel applications. They are a method of synchronizing your database with your application’s codebase in different environments.
Your application changes over time and requires changes to the structure of your database or the creation of tables. Migrations prevent this process from becoming manual, costly, and error-prone.
Every transfer file has a time stamp, providing a complete record of all changes made in the order in which they were made. This makes it easier to roll back to earlier states or apply changes gradually.
Moving a website software application developed in CodeIgniter into Laravel 10 requires careful planning, execution, and testing. This is typically done to benefit from Laravel’s contemporary architecture, extensive features, and thriving community support.
Before delving into Laravel’s 10 migration steps, it’s crucial to understand the reasons why you want to shift from CodeIgniter to Laravel:
- Modern Architecture: Laravel uses a modern PHP framework architecture, following the MVC (Model-View-Controller) pattern more strictly.
- Rich feature set: Laravel comes with built-in features like Eloquent OrM, Blade templating engine, authentication, and much more.
- The community and support: Laravel has a large active community and vast documentation.
Planning the Migration Process
1 Assess Your Current Application
Check out all your CodeIgniter application’s features, functions, and third-party libraries.
Assess each module’s complexity level to determine the amount of work required to move.
Find all dependencies and make sure they’re suitable for Laravel or locate alternatives compatible with Laravel.
2. Set Up a New Laravel Project
Begin by installing a brand new Laravel project with Composer.
Configure Environment Create your `.envFile with the appropriate settings, including credentials for the database.
3. Database Migration
Schema Conversion: Convert your database schema to work with Laravel migrations. You can use tools like Laravel Schema Converter or manually create the migration files.
Eloquent Models: Define Eloquent models for your database tables. Make sure that connections (one-to-one or one-to-many) are established correctly.
4. Route Mapping
Routing in CodeIgniter: CodeIgniter uses a straightforward routing system that defines routes in `application/config/routes.php.`
Routing within Laravel: Laravel uses a more flexible and effective routing system. Create routing in `routes/web.php file.
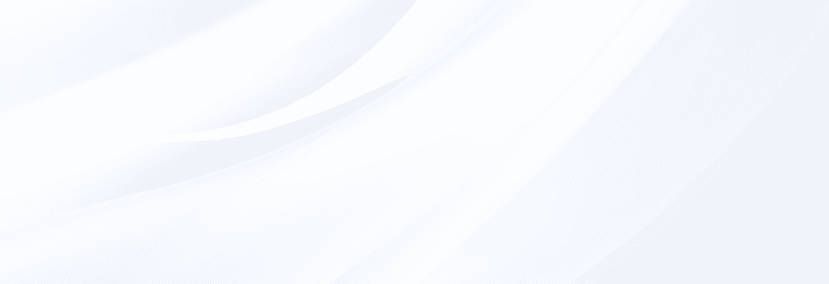
Experience Superior Web Solutions with Our Leading Laravel Development Company!
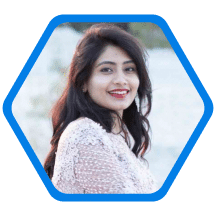
Pooja Upadhyay
Director Of People Operations & Client Relations
Step By Step Guide For Laravel Migration
Step 1. Migrate Configuration
Config Files: Transfer configuration settings from the CodeIgniter application/configdirectory to Laravel’s configuration directory. This includes email settings, database settings configurations, and any other customized configurations.
2. Migrate Controllers
CodeIgniter Controllers: In CodeIgniter, controllers are found in `application/controllers`.
Laravel Controllers: Laravel allows you to create controllers with artisanal commands.
The logic is transferred from CodeIgniter controllers into Laravel controllers. It should be adapted to
Laravel’s response and request handling.
3. Migrate Models
CodeIgniter Models: Found in `application/models`.
Laravel Models: Create models using artisan commands.
Transfer the logic for handling data onto Laravel models, using the Eloquent ORM features.
4. Migrate Views
CodeIgniter Views are located in `application/views`.
Laravel Views: Use Blade templating engine for Laravel views. Place your view files in the resources/views folder and transform them into Blade syntax.
5. Migrate Libraries and Helpers
CodeIgniter Libraries and Helpers are found in `application/libraries` and `application/helpers.`
Convert custom libraries into Laravel aid functions or services. Service classes can be created and then bind them to the Laravel service container.
Testing and Debugging
Unit tests crucial functions to ensure they function as expected within the framework that is being developed.
Extensive manual testing of the whole application is performed to discover any issues or contradictions.
Use Laravel’s debugging tools, such as Laravel Debugbar and the built-in logging, to resolve and troubleshoot problems.
Optimization and Final Adjustments
Performance Optimization: Improve the performance of your Laravel application by setting caching and optimizing queries to databases, as well as using queues for background tasks.
Improvements in Security: Implement Laravel’s built-in security features, such as CSRF protection input validation, input validation, and authentication systems.
Deployment: After testing, install the Laravel application in your production environment. Make sure you have a backup plan for any problems that may occur after deployment.
Post-Migration Considerations
Training: If your team is unfamiliar with the Laravel framework, provide workshops or training to get them up to speed using the new framework.
Documentation: Change your project’s documentation to reflect the changes made during the migration process.
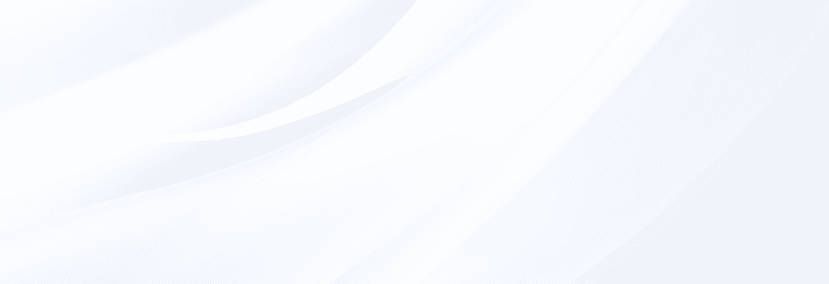
Best Practices for Writing Laravel Migrations
Apart from using Laravel Framework best practices for creating Laravel migrations, it is essential to follow best practices to ensure that your migrations run smoothly and are maintainable and scalable. Here are some of the best ways to think about it:
Utilize the up-and-down method
The migration files should include an up method that defines the schema changes that need to be made and a down method that reverses the modifications. This ensures that your data migrations are reversible, crucial to keeping data integrity intact and enabling rollbacks.
Use descriptive names
Use descriptive names: Name your migrations in a way that makes it easy to comprehend the changes made in the schema. For example, instead of create_table, use create_users_table or add_email_column_to_users_table.
Make use of schema builders
Use Laravel schema builder because it offers the most expression. The Laravel schema builder has a syntax that is too easy to read compared to plain SQL statements. This makes your migrations manageable and more straightforward to comprehend and read.
Do not use direct SQL queries
Do not use direct SQL queries when preparing your migrations, as they are difficult to understand, read, and maintain. Instead, you should use the schema builder to create the schema changes if you want to make them.
Define indexes
Create an index in your database tables to boost performance and speed up query execution. Add indexes to frequently used columns and avoid making unnecessary indexes since they could delay write operations.
Utilize foreign keys
Use foreign keys to ensure reference integrity between tables. This can help keep data consistent and to avoid the possibility of records being lost.
Simple
Make your migrations as easy as possible by focusing only on one task for each migration. This helps you manage and comprehend your migrations and prevents problems caused by complicated migrations.
Utilize default values
Set standard values for your columns so that your application can handle null or missing values. Use the default approach the schema builders offer to set default values for columns.
Data seeding
Use data seeding to fill your database with initial data. Data seeding generates initial table data, such as roles, users, permissions, standard settings, and configurations.
Use timestamps
Use timestamps to create and update columns in your tables automatically. This makes tracking changes and performing audits on your data more efficient.
Utilize database transactions
Use transactions in your database to ensure that your data migrations are entirely independent and that neither all nor none of the processes are carried out. This ensures that your database is constant and prevents data corruption.
Check your data migrations
Thoroughly to confirm they function as you expect. Utilize Laravel’s built-in testing framework to create tests that verify the status of your database before and after the migrations have been executed.
Version your migrations to track changes to your database’s schema over time. Use a program like Git to create versions of your migrations and track changes.
Utilize environment:
Specific configurations to ensure that the migrations work across different environments, including development, staging, or production. Use Laravel’s specific configuration files for your environment to specify other settings for database connections in various environments.
Clean code:
When working on migrations, write clear and understandable code. Use clear and concise variables and method names, observe codes and guidelines, and avoid duplicate code.
Following these additional guidelines will ensure that Laravel migrations are safe, maintainable, and effective and that schema changes to your database are carried out correctly and safely.
Advanced Techniques For Laravel Migrations
Utilize the raw SQL queries
Although the Laravel Schema Builder provides a convenient method of creating columns and databases, you often require raw SQL queries in more advanced situations. The DB: statement() technique to run raw SQL queries inside the migration file.
Use the “change”() method
It is available in Laravel’s Schema Builder, which provides the change() method that lets you modify an existing column within tables. This method can alter the data type and length or change column attributes.
Use foreign keys and indexes
Use foreign and index keys to enhance your database’s performance and ensure data integrity. Laravel’s Schema Builder can create indexes and foreign keys in the migration file.
Utilize database views
Use the database view to build virtual tables that mix the data from various tables. This is useful for reporting or use cases in which you must combine data from different tables into a query.
Customize your migration paths
Laravel’s data files are saved by default in the directory database/migrations. But, when you execute the migrating command, it is possible to specify a customized migration path using the -path option. This can be helpful when you have several modules or programs that contain migration files.
Utilize migration events
Laravel provides several migration events that you can use to trigger custom codes whenever certain events during migration occur. For example, you could use the events before and after to run custom code before or after the migration.
Utilize the command migrate:fresh: The command “migrate:fresh” is an even more powerful refresh version. It will delete the entire database and then re-run migration, starting from the beginning. This helps reset the database to a pre-determined state while developing or testing.
Utilizing these advanced methods when you’re working on Laravel. In such a way, you’ll be able to develop more powerful and effective schema changes to data, making your application more adaptable and reliable.
Is Laravel Migration Necessary?
Laravel migration isn’t required for Laravel application development but is highly recommended for migration. Migrations offer a simple method of managing changes to the database schema over time. They also assist in ensuring that your database schema is standardized across different platforms.
Without the migration, you have to manually alter the schema for your database every time you
update your application’s data model. This could be prone to errors and time-consuming, significantly when your application expands in complexity and size.
They also allow you to reverse changes to your database schema in case of a problem. This is especially crucial when working in production environments, as you must ensure your application is solid and reliable.
Although you can create a Laravel program without migration, it’s recommended that you utilize it to handle modifications to the database schema over time. This can ensure that the application is stable and expandable as it develops.
Migrations in Laravel provide a simple and effective method for controlling changes to your database schema over time, ensuring your application’s stability and capacity as it expands.
Conclusion
Following the advanced techniques for creating and controlling Laravel changes, you can develop efficient schema updates to consistent databases across various environments.
Although they aren’t essential for creating a Laravel application, they are highly recommended to ensure stability, durability, and reliability.
Utilizing Laravel migrations will save you time and effort when managing your database schema, allowing you to concentrate on developing your application’s core features.
Transmitting from CodeIgniter to Laravel 10 is a lengthy process that takes days to weeks, depending on the software’s size and capabilities. Though it requires proper planning and implementation, the advantages of modern technology, enhanced features, and active participation from the community make it worth offering a seamless and robust experience during the migration process.
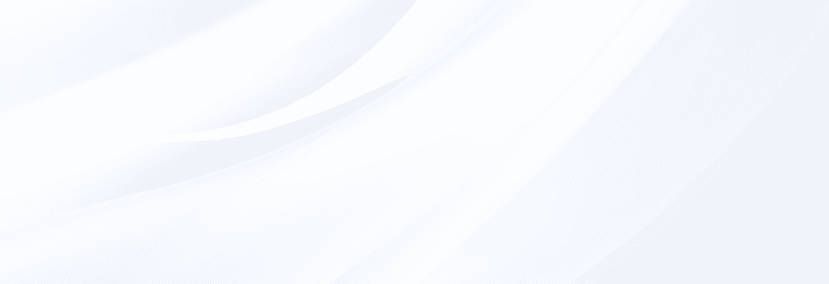
Ready for Excellence? Hire an Expert Laravel Developer for Your Project!
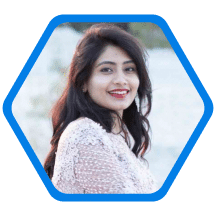
Pooja Upadhyay
Director Of People Operations & Client Relations