React app development is simple. Once registered with React, all that remains to complete your app is to provide some basic details about it, such as its name and description, version numbers, etc. Once submitted, you’ll be presented with a list of software to use on your project; React’s basic principle involves building your user interface using JavaScript XML before using React to turn into DOM nodes in its server-side applications.
Experienced developers already understand what React is; even novice web developers probably encountered and heard of React for front-end development numerous times during their studies and may already know its use, features, benefits, and drawbacks.
Are You Building a React App? Developing React projects is a complex multi-step process that includes setting up a build system, a basic directory structure, and an app that converts modern syntax to code that any browser can read. It is an important step that must be addressed when developing React apps.
Create-React-App contains the complete set of JavaScript packages (basic testing, linting code transpiring, and building systems) needed to build React applications quickly and successfully.
Create-react-app includes an instant reload feature that refreshes your site whenever you modify code while developing. Simply put, this allows you to build your background quickly and start writing code immediately by creating elements and structuring directories quickly and efficiently.
React at a Glance
React, which is maintained and developed by Facebook, is an open-source JavaScript library to create user interfaces and UI components. Popular for its scalability and flexibility, React has become a preferred choice for developers working on single-page applications as well as complicated web interfaces. The component-based architecture allows for reusability and ease of maintenance, making it a great solution for smaller-scale and large-scale projects.
Significance of Create-React-App
Although React makes it easier to do UI development, starting with a new app typically involves configuring a variety of settings, tools, and dependencies, a process that can be lengthy and prone to errors. This is where Create-React-App (CRA) can help streamline the initial set-up process and eliminate manual configuration requirements. It’s a command line tool that allows developers to build an entirely new React application using one command, which can save valuable time and effort.
Simple and Fast with Create-React App
One of the major benefits of Create-React-App is its ease of use. Whether you’re an experienced developer or just beginning with React, CRA abstracts away the intricate build configurations so you can concentrate on the most important thing: writing code. By a single command, the Create-React App creates a new project with a sensible default that includes a building server for development, scripts to build, and a well-organized structure for the project.
The speed with which the Create-React App operates is simply incredible. In just minutes, you will be able to have a fully functioning React application in operation. It’s beneficial for developers seeking to rapidly prototype ideas and test concepts or participate in the process of iterative web app development. Create-React App speeds up the beginning of an application, allowing developers to begin coding and innovating without the burden of complex set-ups.
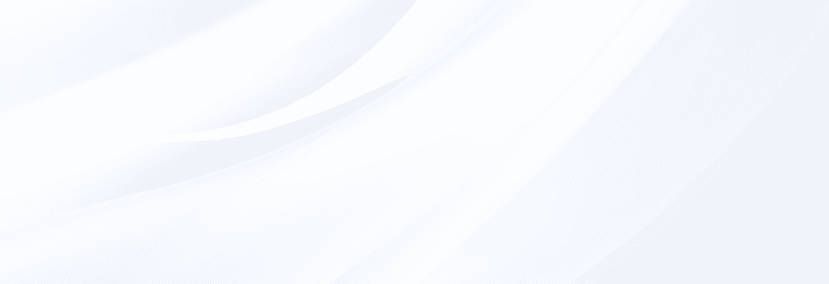
Leverage the best web development technologies – React to create scalable Enterprise Applications. Also, hire React Developers from Addweb on a Contract.
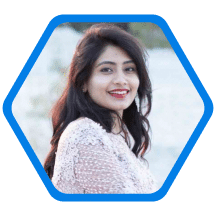
Pooja Upadhyay
Director Of People Operations & Client Relations
How to create a React App with Create-React Quickly
Node.js is a JavaScript runtime platform that lets users run JavaScript code without an internet browser. It contains everything required to run JavaScript applications, including a virtual machine, the package manager (npm), and an array of modules. npm, abbreviated as Node Package Manager, is a command-line tool used to manage packages and dependencies of Node.js projects.
Before you proceed, ensure that Node.js and npm have been installed on your computer. You can download and install Node.js from the official website (https://nodejs.org). NPM is included in Node.js, and after you’ve installed Node.js installed, it will also be accessible in the same way.
To confirm your installation Node.js as well as npm you need to open your Command-Line Interface (CLI) and execute these commands
Copy codes
Node -v
npm -v
If both commands show a version number (e.g., v14.17.0 for Node.js and 6.14.13 for NPM), It indicates that Node.js and npm are thriving on your computer.
Basic Understanding of Command-Line Interface (CLI)
Create-React-App is essentially a command-line tool, meaning that you’ll need the CLI to communicate with it. So, having a basic knowledge of the command-line interface is vital.
Learn the most common CLI commands, such as navigation of directories (cd), listing the contents of a directory (ls or dir), and making directories (mkdir) as well as running commands (npm or npx.). Knowing the commands you can use to move around using the CLI can greatly ease the creation and management of React applications using Create-React.
If you ensure the Node.js and npm have been installed on your system and understand the command line GUI, you’ll be prepared to begin creating an original React app with Create-React in only five minutes.
Install Create-React-App
Installing the Create-React-App package is the first step to quickly building a new React application. This is done by using the npm package manager, also known as an extension of the Node Package Manager, to enable globally the Create-React-App application on your system.
- Understanding npm
NPM is a package manager for JavaScript that is included with Node.js installation. It allows React developers to find, share, and utilize code packages and easily manage project dependencies. NPM plays a significant part in the JavaScript ecosystem by making it easier to manage adding, updating, and removing software from the project.
- installing packages globally Using NPM
Before we get into the installation process of Create-React-App, it’s crucial to understand how npm handles global installation. If a package is installed globally, it’s accessible across the entire system, and the executable commands are accessible from any directory within the Command-Line Interface (CLI).
To install a global package with npm, this structure of commands is employed:
GoCopy code
NPM install with -g
In this case, the flag -g flag indicates the installation is global and is replaced by an identifier for the application to be installed worldwide.
Installing Create-React-App Globally
To install Create-React-App all over the world, you must open your command-line console and execute this command
luaCopy codes
npm install -g create-react-app
This command instructs npm to create the create-react-app package on a global basis on your computer. Its “g” flag assures that the package is accessible across the entire system, allowing you to execute this create-react-app command from any location in the CLI.
When the installation process is completed, you can access the Create-React App command-line tool, which allows you to quickly create new React applications with already defined settings and configurations.
Create a New React App
Creating an entirely new React application with Create-React is an easy procedure requiring only one command to establish an organized project structure with reasonable defaults. Let’s explore the process of creating a brand new React app and look at the final structure of the project, as well as the files.
- Using Create-React-App to Set Up a New Project
Once the Create-React App is installed worldwide on your system, you can start creating a new React application using the following command from your Command-Line Interface (CLI):
luaCopy Code
npx create-react-app my-react-app
This is where the my-react app will be the title you could replace with the name of your project. The command npx command can be utilized to run Create-React-App without the need to install it and ensure that the most recent version is installed in the project.
Create-React App will download the required packages and create the project structure according to the best guidelines and practices.
1. node_modules: This directory houses all dependencies of the project. The create-react-app command automatically creates it. There is no need to manage these dependencies manually because npm handles this.
2. Public: The directory contains the public assets of your application, like HTML-based files and images and the Favicon. Index.html is the index.html file that is the entry point to start your React application.
3. Src: It is the Src directory where the source code of your React application is located. It contains the core application files, including component styles, assets, and others.
- index.js: This file is the entry point to React. React application. It converts the root component to its root element in the DOM as specified within the public/index.html file.
- App.js: The default component file which acts as the primary component of your application. You can modify or substitute this component to the requirements of your project.
- App.css: The default stylesheet that is used for this app component. You can also add more styles or make separate CSS files if needed.
4. package.json: This file records metadata for the application and all its components. Additionally, it contains scripts for performing different tasks, including beginning the development server and creating production versions.
Navigate to the Project Folder
Accessing the project folder with commands is a crucial ability when working on projects for software development. When making a new React application using Create-React-App, entering your project directory is the first step following the initial configuration. We’ll look at utilizing the command line to switch directories, then enter your newly-created React App folder.
- How to Use the Command Line to Change Directories
A command line interface (CLI) is an effective and efficient method to interface with your computer’s file system. The Cd command, “change directory,” is used to navigate between different directories in your file system.
Here are some of the most basic Cd commands:
- To change to an existing directory area:
BashCopy Code
CD folderName
- To be able to move into an existing directory in a certain direction:
BashCopy Codex
cd path/to/your/directory
- To go up a step in the tree of directories:
BashCopy Code
cd ..
- To go directly into your directory of home:
BashCopy Code cd ~
- Move to the Newly Created React App Folder
After you’ve created a new React application using Create-React-App, the program automatically creates an appropriate folder that bears the project’s name. If you’ve given your project the name “my-react-app,” you can access the folder by using these commands:
copy bashCopy codes
cd my-react-app
This command instructs the line of command to switch the currently used working directory to a folder called “my-react-app.” When you execute this command, it will be inside the project folder, and any subsequent actions or commands are performed in the context of the React application.
Accessing the project folder is essential because it lets you modify and access your source code, manage dependencies, and run various commands related to the building and development processes. When installing your development server and examining the default React application, staying in this project directory is vital to ensure a smooth development experience.
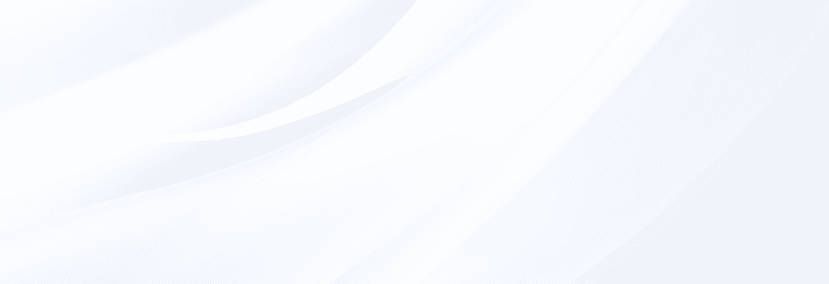
Run the Development Server
After your newly created React application is running, the next important step is to launch the server development. The server lets you test and communicate with your React application during creation. Create-React-App makes this process easier by allowing you to use a simple command.
- Start the Development Server Using a Simple Command
To begin your development server, you need to open the command line interface (CLI) by navigating to the folder for your project if you’re not there. After that, you can run this command
copy bashCopy codes
NPM begin
The command is located within the “scripts” section of the package.json file, generated by Create-React-App in the set-up of your project. It starts the development server, creates your React app, and then displays it in the default browser of your choice.
- Explanation of What the Development Server Does
The development server has different purposes during the development stage of the React application.
1. Live Reloading: It monitors for any changes to your source code and then automatically refreshes the application within the browser, giving you an immediate preview of your modifications.
2. Error reporting: When there is a syntax error or other problems in your code, the development server will show error messages on the console to aid in the quick identification of and resolution.
3. Localhost Environment: This server executes your React app in local development environments. By default, it uses http://localhost:3000, allowing you to access and test your application locally.
- How to Access the App in the Browser
After the development server has been properly started, you can access the React application through your browser on the internet and going to the following URL:
arduinoCopy code
http://localhost:3000
It is also the address that developers use for their development servers. You should be able to see your React application running in the browser. Any changes in the code source will be immediately reflected when you save your files.
The development server’s operation is an important element of the React web application development process. It is a highly dynamic and efficient platform for programming or testing and enhancing your React application before moving it into an environment for production. In the next steps, we’ll look at the default React application and make swift adjustments to comprehend better how to develop.
Explore the Default App
After successfully opening the project folder, the next step in creating a brand new React application with the Create-React App is to investigate the default React application that is generated automatically. The exploration will provide insight into the app’s structure and other important files created by the Create-React App.
#Overview of the Default React App
Create-React-App creates an initial React structure for applications that follow best practices and support the modular and scalable web development method. The main elements in the app’s default structure are:
1. Public Folder:
- index.html: The main HTML file which acts as the entry point to React. React application. It also contains an
With an ID with a “root” where the React application is mounted.
2. Src Folder:
- index.js: The primary JavaScript file in which the React application is launched, and the main component is rendered. The file is loaded with ReactDOM, the ReactDOM library, to render the app component to the identified HTML element.
- App.js: The default component file, which is used to represent the core application. The app.js component is the basic structure of the application with the default header and a “Welcome to React” message. Developers can modify and extend this component to develop their applications.
- App.css: The default stylesheet of this app component. It provides basic styling rules that are applied in the standard content of App.js.
3. Node Modules:
- The node_modules folder houses all dependencies of the project. Create-React-App will automatically install the required software listed within the package.json file during the initialization of the project.
4. Package.json:
- Its package.json file details the project and defines its dependencies, scripts, and other configurations. It acts as the central configuration file for the project.
5. Other Files:
- Service worker files (serviceWorker.js, registerServiceWorker.js): These files are related to Progressive Web App (PWA) functionality, enabling features like offline caching.
#Brief Explanation of Important Files
1. index.html:
- The HTML structure is comprised of
In which the React application is to be in the area where React will be.
- Meta tags and hyperlinks to the favicon as well as other sources.
2. index.js:
- Imports React and ReactDOM libraries.
- It imports the component from the App component.
- Renders this Application component to the HTML root element.
3. App.js:
- The functional React component serves as the core application.
- It has a default header and is accompanied by a “Welcome to React” message.
- The basic design of the React component.
4. App.css:
- It contains basic styles that are applied in the standard content of App.js.
- The application can be expanded and redesigned to create a unique style.
By looking through these default files, developers can understand how the React application is designed, begin adjusting, and build on this foundation to satisfy their requirements. In the next stage, we’ll modify the app and observe the app’s immediate effect on your browser.
Make a Quick Change
Once we’ve become familiar with the basic structure used by the React application developed by Create-React App, Let’s begin the thrilling process of making a swift modification to the application. This hands-on learning experience will show the simplicity and speed of the development process in React.
- Demonstrate How to Make a Simple Change in the App:
Let’s alter what is the standard “Welcome to React” message within App.js. App.js file. Navigate to this src/App.js file in the editor you prefer to use. Editors include Visual Studio Code, Atom, or any other editor.
In App.js, within App.js file, find the following code lines:
JsxCopy code
return (
Welcome to React
);
Let’s change the text within the
tag to be more personal. For instance, you could modify it to:
jsxCopy Code
Hello, React Enthusiast! Let’s create amazing things together.
- Save and See the Instant Update in the Browser:
After you have made this modification After making this simple change, save your App.js file. Let’s now see the immediate update in the browser.
1. Open your command line interface (CLI) and make sure you’re in your project’s folder.
2. Start the server for development by using this command.
BashCopy Code
NPM begin
This command initiates the development server, and you should see an output indicating that the app is running and the server is listening on a specific port (commonly, http://localhost:3000).
1. Open your web browser and navigate to the provided address (http://localhost:3000). You’ll see the latest message indicating the modification you made to App.js. App.js file.
The browser will automatically refresh with the new content without the need to refresh manually. Live reloading is a feature that enhances developers’ experience, allowing them to monitor the effects in real time.
1. In addition, make sure to start the public/index.html file and try altering the name of the web page. Watch how the modification is reflected instantly within the browser.
By making these small adjustments and observing the web browser’s immediate updates, you can experience firsthand the speed and responsiveness of the development platform provided by the Create-React App. This development method allows developers to play around in the lab, create prototypes, and refine their applications quickly.
The Key Takeaway
In conclusion, our study of creating a completely new React application using Create-React-App has demonstrated the incredible speed, simplicity, and interactivity this tool can bring to the mobile app development process. From installing Create-React App worldwide and accessing the project folder to making a swift edit and seeing immediate updates in the browser, The seamless workflow provided by Create-React App speeds up the initial phases of React development.
This allows developers to build efficient and reliable applications without the burden of lengthy set-up procedures. When we begin our React development process, React’s versatility and the convenience of Create-React-App provide the base for an efficient and enjoyable coding experience. With the new knowledge developers have gained, they can develop and extend your React initiatives with confidence and a sense of creativity.
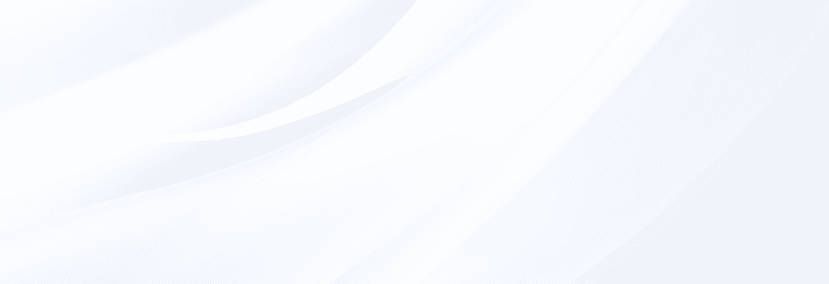
Do you have questions about React development? Hire our React developers for various services for the technology.
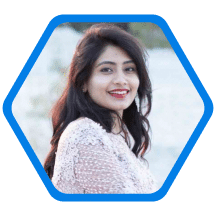
Pooja Upadhyay
Director Of People Operations & Client Relations