Are you looking to become a Flutter master and seeking the finest Flutter course? Flutter revolves around widgets, the fundamental building blocks of your app. In the Flutter framework, devices are categorized into two main types: Stateless and Stateful.
Let’s dive into the concept of “state” in widgets. Imagine a lightbulb in your app that you want to switch on and off. The state of the lightbulb being either on or off represents its current condition. In more technical terms, the state is the information that can change over time, influencing how your widget appears or behaves.
Stateless Widgets
In Flutter, Stateless Widgets form the foundation of stability and efficiency in constructing user interfaces. Understanding their characteristics and best practices is essential for developers aiming to create predictable and static components within their applications.
Understanding Stateless Widgets:
Key Characteristics:
Immutability: Once constructed and rendered, a stateless widget’s properties and appearance remain unalterable. This immutability ensures consistency throughout its lifecycle.
Static Behavior: Stateless widgets exhibit static behavior, making them ideal for representing content that does not require updates based on user interactions or external events.
Efficiency and Performance: Stateless widgets are lightweight and efficient. Lacking mutable states, they do not need to track changes or trigger rebuilds, contributing to optimal performance in Flutter applications.
Anatomy of a Stateless Widget:
- Class Declaration: Extends the Stateless Widget base class.
- Constructor: Defines parameters for customization during widget initialization.
- Override the build Method: Describes the UI of the widget and returns a widget tree for rendering.
Use Cases for Stateless Widgets:
- Static Content: Stateless widgets are suitable for components with content that remains constant and doesn’t require dynamic updates.
- Predictable Rendering: When UI elements demand a predictable and deterministic rendering approach, stateless widgets offer stability.
- Simplicity: For simpler UI component based design without intricate state management needs, stateless widgets are an ideal choice.
Best Practices:
- Immutability Awareness: Embrace the immutability of stateless widgets to ensure consistency in appearance and behavior.
- Static Content Representation: Leverage stateless widgets for representing static content, such as text, icons, or images.
- Efficient Rendering: Utilize stateless widgets in scenarios where optimal performance and efficient rendering are priorities.
Stateful Widgets
In Flutter development, Stateful Widgets serve as dynamic powerhouses, enabling developers to create interactive user interfaces that respond to user interactions and external events. Understanding the characteristics and best practices associated with stateful widgets is crucial for building responsive and adaptable components within Flutter app development agency.
Understanding Stateful Widgets:
Key Characteristics:
- Mutable State: Unlike stateless widgets, stateful widgets possess mutable states that can be modified and updated throughout their lifecycle.
- Dynamic Behavior: Stateful widgets can adapt and change based on user interactions, external events, or modifications to the underlying data.
- Rebuilds with State Changes: Changes to the mutable state trigger a rebuild of the widget, ensuring that the updated form is reflected in its appearance.
Two-Class Structure:
- StatefulWidget: Represents the stateful widget itself and manages the lifecycle of the corresponding State class.
- State Class: Resides within the StatefulWidget and holds the mutable state, containing data and logic that can change during the widget’s lifecycle.
Use Cases for Stateful Widgets:
- Dynamic UI Elements: Stateful widgets are ideal for creating UI elements that require dynamic changes and adaptations based on user interactions.
- Interactive Components: Components like counters, form inputs, or animations benefit from the dynamic behavior provided by stateful widgets.
- Reactive User Interfaces: When UI elements need to react to changes in application state or user input, stateful widgets offer the necessary flexibility.
Best Practices:
- Mutable State Management: Effectively manage mutable states within the state class and use setState() for state updates to trigger rebuilds.
- Interactive UI Elements: Employ stateful widgets for UI components requiring user interactions, ensuring a responsive and dynamic user experience.
- Rebuild Optimization: Be mindful of optimizing rebuilds to maintain performance, especially in scenarios with frequent state changes.
Stateful Widgets Explained:
Stateful widgets represent a specific type of widget in Flutter capable of maintaining a mutable state. This implies that they can change or update their appearance and behavior over time. The primary responsibilities of a Stateful Widget include managing the changed state based on its previous form and ensuring an updated view of the user interface. These widgets find their utility when building parts of the UI that require dynamic updates in response to various stimuli like user interactions, network requests, animations, or changes in data.
Lifecycle of a Stateful Widget:
createState(): This is the initial method called when a stateful widget is introduced into the widget tree. It creates an instance of the State class associated with the device.
Mounted: This is a boolean value that turns true when the buildContext is assigned to the widget.
initState(): Called once after the State object is created or before the widget is built; this method is used for initializing data or performing setup operations. It runs only once during the widget’s lifecycle.
didChangeDependencies(): This method is called just after initState() and can be called multiple times. It triggers whenever the dependencies of the State object change, like when a StatefulWidget depends on an InheritedWidget.
Build(): Responsible for returning the widget’s user interface, this method rebuilds when setState() is called, typically due to changes in the widget’s state, parent widget, or device orientation.
didUpdateWidget(): Called if the parent widget changes and triggers a rebuild of the stateful widget; this method is invoked whenever the Stateful widget is updated with a new instance of itself.
setState(): This method signals that the widget’s internal state has changed, requiring a rebuild. Upon calling setState(), it schedules a call to the build method.
Deactivate(): This is called when the widget is popped but might be reinserted before the current frame change is finished. This occurs when the device is no longer visible on the screen.
Dispose(): This method is called when the widget is permanently removed from the widget tree, and it serves to clean up resources.
Understanding the lifecycle of a Stateful Widget is crucial for effective Flutter development, enabling developers to manage dynamic UI components with precision and responsiveness.
What is a Dynamic Widget?
A dynamic widget in Flutter refers to a widget that can be generated and modified during the runtime of a mobile application, providing increased customization and interactivity in user interfaces. Examples of such widgets include ListView.builder, GridView.builder, and Wrap, which efficiently reduces code redundancy in Flutter applications.
Dynamic widgets simplify the creation of intricate and data-driven UI elements such as buttons, text fields, and sliders. They enable developers to easily customize and update widgets on-the-fly, offering a variety of interactive UI components. Here are a few methods to create dynamic widgets in Flutter:
1. Using the Builder Widget:
The Builder widget employs a function as its child, and this function is invoked whenever the widget requires rebuilding. This function can dynamically generate the widget’s content at runtime.
2. Using the StatefulWidget Class:
The StatefulWidget class allows the creation of widgets with mutable state. The state of a widget can be altered during runtime, triggering the widget to be rebuilt.
How Are Dynamic Widgets Useful in Creating Interactive Flutter Applications?
Dynamic widgets prove useful in several scenarios, including:
Creating widgets based on user input: Designing a dynamic widget that displays distinct text fields based on the user’s selection from a dropdown menu.
Creating widgets based on data: Developing a dynamic widget that exhibits a list of items retrieved from a database.
Creating widgets responsive to changes: Designing a dynamic widget that adapts its appearance when the user’s device orientation changes.
The Benefits of Using Dynamic Widgets in Flutter:
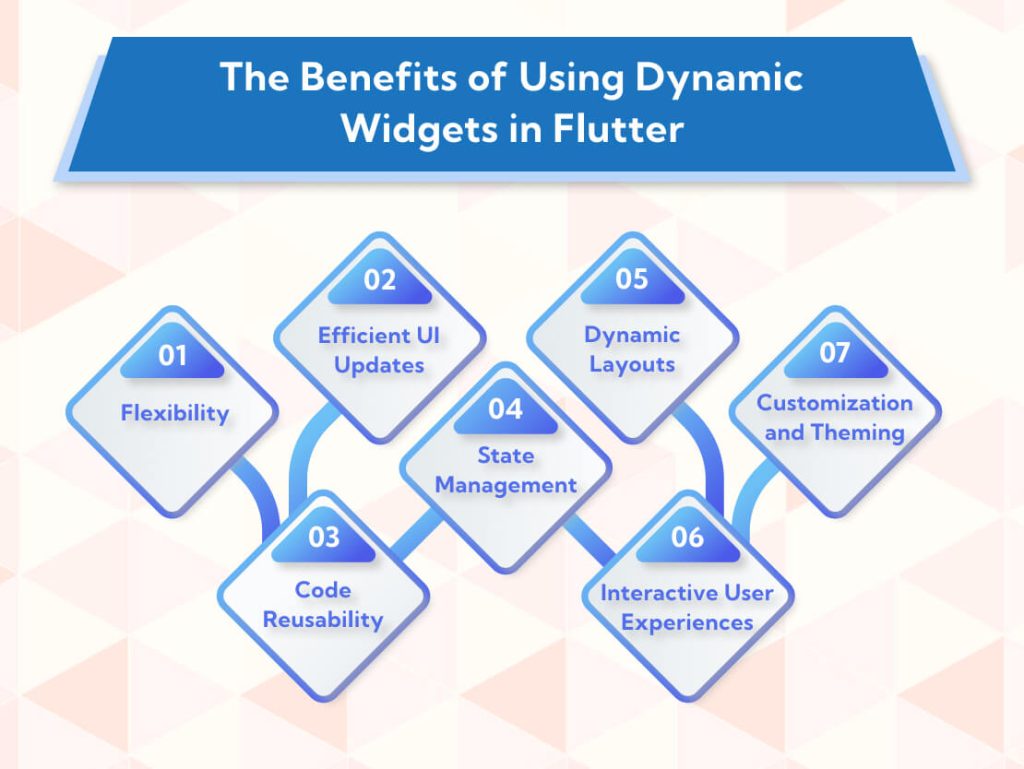
1. Flexibility:
Dynamic widgets enable the creation of adaptable user interfaces that respond to dynamic data or user interactions, fostering highly interactive and personalized applications.
2. Efficient UI Updates:
Flutter’s declarative UI framework efficiently updates only the necessary parts of the user interface when changes occur. Dynamic widgets facilitate targeted updates based on data changes, enhancing performance and reducing unnecessary UI updates.
3. Code Reusability:
Dynamic widgets support the creation of reusable components that can be easily configured and reused throughout an application. Encapsulating dynamic behavior within widgets helps avoid code duplication and promotes a modular and maintainable codebase.
4. State Management:
Dynamic widgets play a crucial role in managing and updating the state of a hire dedicated Flutter developer. Combining dynamic widgets with state management techniques like Provider, Riverpod, or BLoC allows efficient handling and propagation of state changes throughout the UI.
5. Dynamic Layouts:
Flutter’s widget system allows the creation of dynamic layouts that adjust based on available screen space or device orientation. Dynamic widgets enable the development of responsive designs that adapt to different screen sizes and orientations, ensuring a consistent user experience across devices.
6. Interactive User Experiences:
Dynamic widgets empower developers to build interactive and engaging user experiences. These widgets respond to user input, animate between different states, or dynamically load data from external sources, enhancing the interactivity and richness of an application.
7. Customization and Theming:
Dynamic widgets facilitate easy customization of the appearance and behavior of UI elements based on various factors. Whether dynamically changing colors, fonts, or layouts, these widgets allow the creation of highly customizable and themeable user interfaces.
A Simple Implementation Example of Using Dynamic Widgets in a Flutter App:
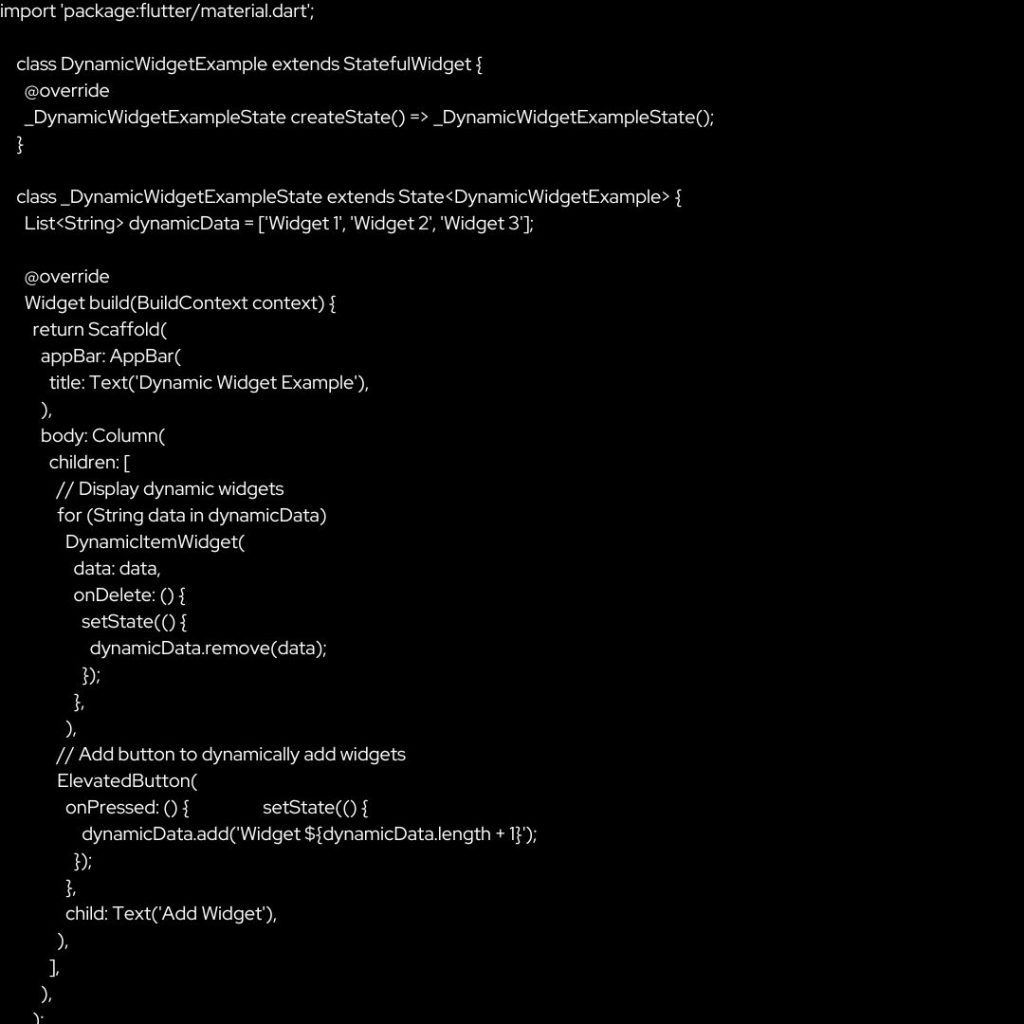
Conclusion
By understanding the nuances of stateful and stateless widgets and implementing these optimization strategies, developers can create Flutter applications that deliver exceptional performance in 2024 and beyond. Choose the right widget type based on the specific requirements of each component, and employ best practices to ensure a smooth and efficient user experience.
Frequently Asked Questions (FAQs) about Stateful and Stateless Widgets in Flutter:
Converting a Stateful Widget to a Stateless Widget involves refactoring your widget code to eliminate mutable state and update methods. You’ll need to remove the state management logic and transform the widget into an immutable representation.
The state of a Stateful Widget in Flutter refers to its mutable data that can change over time. This data is stored in the associated State object and influences the appearance and behavior of the widget.
Yes, it is possible to place a Stateful Widget inside a Stateless Widget in Flutter. This combination is useful when you want to incorporate dynamic and interactive elements (Stateful) within a predominantly static UI structure (Stateless).
Absolutely, a Stateless Widget can encapsulate a Stateful Widget in Flutter. This allows you to embed dynamic and interactive components within a primarily static part of your user interface.
In Flutter, you call a Stateless Widget by instantiating it using its constructor. Once instantiated, you can include it as a child in the widget tree, and it will render its immutable UI representation.
Generally, a button in Flutter is implemented as a Stateless widget because its appearance remains constant once built. However, the interaction and behavior of the controller may be managed by a Stateful Widget to handle dynamic changes, such as button presses.